We use cookies to make your experience better. To comply with the new e-Privacy directive, we need to ask for your consent to set the cookies. Learn more.
VirtualType of Magento2 DI
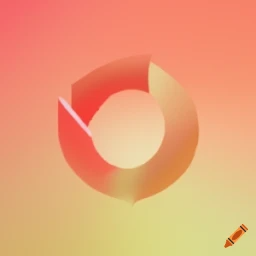
In Magento 2, a VirtualType is a type of object that is created and configured using the dependency injection (DI) mechanism.
A VirtualType is not a concrete class, but rather a way to define a set of arguments and configurations that can be used to generate an instance of an object on-the-fly. This can be useful when you need to create an object that requires a complex set of dependencies or configuration options, but you don't want to create a concrete class for it.
To create a VirtualType in Magento 2, you first need to define it in the di.xml file of your module.
To define a virtual type, you need to specify the following in the di.xml file:
name: The name of the virtual type. This should be a unique identifier that is used to refer to the virtual type in other parts of the Magento 2 codebase.
type: The class that the virtual type is based on.
shared: A Boolean value that indicates whether the virtual type is a singleton or not. If set to true, the virtual type will be a singleton and only one instance will be created per request. If set to false, a new instance will be created each time the virtual type is requested.
Here's an example:
<virtualType name="MyVirtualType" type="My\Module\Model\MyClass">
<arguments>
<argument name="arg1" xsi:type="string">value1</argument>
<argument name="arg2" xsi:type="string">value2</argument>
</arguments>
</virtualType>
In this example, we define a VirtualType called MyVirtualType, which is an instance of the My\Module\Model\MyClass class. The arguments section allows you to specify any arguments that the constructor of MyClass requires. In this case, we're providing two string values for arg1 and arg2.
Once you have defined your VirtualType, you can use it in your code by injecting it as a dependency. For example:
use My\Module\Model\MyClass;
class MyController extends \Magento\Framework\App\Action\Action
{
protected $myVirtualType;
public function __construct(MyClass $myVirtualType)
{
$this->myVirtualType = $myVirtualType;
}
public function execute()
{
// Use $this->myVirtualType here
}
}
In this example, we're injecting our VirtualType MyVirtualType into a controller. Magento 2's DI system will automatically create an instance of MyClass with the arguments we specified in the di.xml file, and pass it to the controller's constructor.
Using VirtualTypes in Magento 2 can help simplify your code and make it more modular and flexible.